06 - Data Structures: Sets
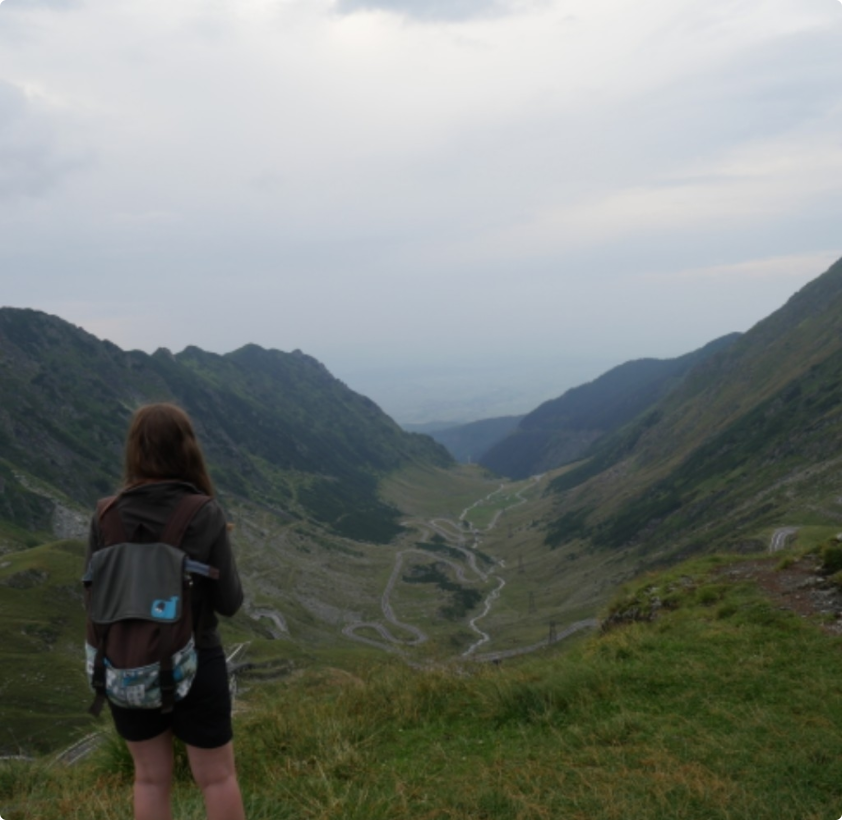
Goals
- learn what are sets and math theory behind it
- learn when sets can be used
- how sets are different from the lists
- get familiar with two implementations coming in JDK:
HashSet
,TreeSet
- get familiar with the most commonly used methods on sets
Tips
How to generate a random number?
To return a random number from range between 0
and 50
(exclusive):
Random r = new Random();
int randomNumber = r.nextInt(50);
Slides
Exercises
Exercise 1
In main
method
- Create a new HashSet of strings, add
banana
,apple
,orange
. - Print content of sets using for loop
- Clear the set.
- Add one more element
grapes
. - Check if set is empty, and if itβs not empty print βI am not empty!β. What is the order of elements after adding them to HashSet?
Exercise 2
Write a static method randomSet
that will return a HashSet
containing a collection of n
unique random numbers in range 0-100.
In the main method iterate over the returned Set and print each number multiplied by 2.
Where n
is a number of random elements in set.
Exercise 3
Change data type in previous exercise from HashSet to TreeSet. What is the difference?
Exercise 4
Write a static method intersection
that will return an intersection of two sets given by parameters. Note - sets given by parameters may not be modified.
Exercise 5
Write a static method union
that will return an union of two sets given by parameters. Note - sets given by parameters may not be modified.
Exercise 6
Write a static method difference
that will return a difference between two sets given by parameters.
Note - sets given by parameters may not be modified.
Exercise 7
Write a static method symetricDifference
that will return the symetric difference between two sets given by parameters.
Note - sets given by parameters may not be modified.
Exercise 8
Create a TreeSet containing integers that will maintain descending order of numbers. Hint: Look at alternative TreeSet constructors.